Python compilers: what they are, best examples, and how to choose one
Python is primarily an interpreted language, but its execution involves both compilation and interpretation. Python code is first compiled into bytecode, which the Python Virtual Machine (PVM) then executes.
Because of this dual nature, some developers mistakenly believe Python doesn’t use compilers. But you can use a compiler to improve performance, optimize execution, or protect your source code.
This article will explain what a Python compiler is, how it works, and the best options available. We’ll also cover each compiler’s key features, strengths, and drawbacks to help you choose the right one for your needs.
What is a Python compiler?
A Python compiler translates Python code into machine code, or an intermediate language, so it can run without an interpreter. This approach differs from traditional interpretation, where the code is processed line by line at runtime.
While Python typically relies on an interpreter, using a compiler offers several advantages:
- Performance improvement – compiled code often runs faster than interpreted code because it’s already translated into machine code, eliminating the overhead of real-time interpretation.
- Standalone executables – some compilers let developers package Python apps as standalone executables, simplifying deployment on systems without a Python environment.
- Code protection – compiling code helps blur the source code, making it harder to reverse-engineer and adding a layer of protection for intellectual property.
How do Python compilers work?
When a Python program runs, it goes through two main steps:
- Compilation to bytecode – the Python compiler first transforms the source code into an intermediate form called bytecode. This bytecode is a lower-level, platform-independent representation that executes more efficiently than raw source code. It’s typically stored in .pyc files within the __pycache__ directory.
- Execution by the Python Virtual Machine (PVM) – the PVM reads the bytecode and translates each instruction into machine-specific operations that the underlying hardware can execute.
Python compilers use two common compilation techniques:
- Ahead-of-time (AOT) compilation – the compiler translates the source code into machine code before execution. This approach reduces startup time since the code is precompiled, but it doesn’t allow for optimization based on runtime conditions.
- Just-in-time (JIT) compilation – the compiler transforms the code into machine code at runtime, just before execution. This lets it optimize based on actual execution patterns, potentially improving performance. But, JIT compilation introduces some overhead since it occurs during execution.
Suggested reading
Whether you’re building dynamic websites, developing AI models, or analyzing large datasets, discover five powerful ways to use Python in this article.
8 best Python compilers
Here are the top Python compilers for different use cases, whether you need to improve performance, convert Python code to another language, or create standalone executables.
1. CPython
CPython is the reference implementation and the most widely used version of the Python programming language. Written in C, it’s the standard for other Python implementations.
When you download Python from the official website, you’re getting CPython.
As an interpreter and a compiler, CPython first compiles Python code into an intermediate bytecode. Its virtual machine then executes this bytecode, ensuring efficient execution while maintaining compatibility across different platforms.
Key features:
- Extensive standard library – includes a complete set of modules and packages, often called “batteries included.” These cover various tasks, from file I/O and web development to data processing and networking.
- Extensibility – integrates with C and C++ through its C API, letting Python developers use existing C libraries and build performance-critical components.
- Cross-platform compatibility – runs seamlessly on multiple platforms, including Windows, macOS, and Unix-based systems.
Pros:
- As the official implementation, CPython benefits from rigorous testing and continuous development.
- Its widespread adoption ensures compatibility with numerous libraries and frameworks.
- Supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Cons:
- Uses the Global Interpreter Lock (GIL), which limits multi-threaded performance by letting only one thread execute Python bytecode at a time.
- May run slower than implementations like PyPy, particularly in CPU-bound apps.
2. PyPy
PyPy is an alternative Python implementation that provides faster execution and improved efficiency. Unlike the standard CPython interpreter, it uses a JIT compiler, which boosts performance by compiling Python code to machine code at runtime.
PyPy is written in Restricted Python (RPython), a subset of Python optimized for interpreter development. This design makes it easier to modify and optimize PyPy itself.
Key features:
- Just-In-Time (JIT) compilation – dynamically compiles Python code to machine code during execution, resulting in faster runtime performance.
- RPython toolchain – enables the creation of efficient language interpreters so developers can experiment with new language features and optimizations.
- Compatibility – supports multiple Python versions, including Python 2.7 and 3.10, making it suitable for various projects.
- Memory efficiency – uses advanced garbage collection techniques, reducing memory consumption compared to CPython.
Pros:
- JIT compilation can significantly improve execution speed, especially for long-running apps.
- The RPython framework lets developers explore and implement new language features with ease.
Cons:
- Some CPython extensions, particularly those written in C, may not work with PyPy, requiring code modifications or alternative libraries.
- The JIT compiler introduces an initial delay as it compiles code paths during execution, which can be a drawback for short-running scripts.
3. Cython
Cython is a programming language that extends Python by letting developers write C extensions. It compiles Python code into C or C++ modules, significantly improving execution speed.
Cython bridges the gap between Python’s ease of use and C’s performance efficiency by enabling direct interaction with C libraries and systems. It supports optional static type declarations, reducing the overhead of Python’s dynamic typing system.
Key features:
- Seamless C integration – allows direct calls to C functions and the use of C libraries within Python code, making it easy to integrate with existing C/C++ codebases.
- Static typing support – provides optional static type declarations, improving performance and enabling early error detection during compilation.
- Enhanced memory management – gives developers fine-grained control over memory allocation and deallocation, optimizing resource usage in memory-intensive apps.
Pros:
- Compiles Python code into highly optimized C code, leading to better performance in computation-heavy tasks like scientific computing and numerical analysis.
- Maintains Python’s readability and simplicity, making it accessible to developers looking for performance enhancements.
Cons:
- Requires explicit memory management, increasing the risk of memory leaks or segmentation faults if not handled carefully.
- Using C-level constructs can make the code more complex and harder to maintain.
- Introduces dependencies on specific C libraries or compilers, which may affect portability across different systems.
4. Shed Skin
Shed Skin is an experimental compiler that translates Python code into optimized C++ code.
It uses implicit static typing to infer variable types and convert Python scripts into C++ programs. These programs can then be compiled into standalone executables or extension modules for integration into larger Python apps.
To enable effective translation, Shed Skin imposes certain restrictions on Python code. For example, variables must maintain a static type throughout usage.
Key features:
- Implicit static typing – uses type inference to determine variable types without explicit type declarations, ensuring smooth translation into C++.
- Extension module generation – lets developers create C++ extension modules that can be imported into larger Python applications, promoting code reuse and integration.
Pros:
- Produces standalone executables that don’t require a Python interpreter, simplifying deployment and distribution.
Cons:
- Supports only a limited subset of Python features, excluding elements like nested and variable argument functions, which require code refactoring.
- Lacks full support for the Python standard library, limiting its usability for some projects.
- Type inference may not scale beyond several thousand lines of code, making Shed Skin more suitable for small programs and extension modules.
5. Nuitka
The source-to-source compiler Nuitka converts your Python code into executables that can run without a separate installer. It’s free, but a commercial plan is available with additional features like encrypted traceback.
During compilation, Nuitka applies various optimization techniques to make your code more efficient, like built-in call prediction, type inference, and conditional statement execution.
Key features:
- Standalone executables – your code and the interpreter are packed into a single file, allowing you to run a Python program in another system without installing dependencies.
- Encrypted tracebacks – secures your traceback outputs to prevent users from reading your application’s code during troubleshooting.
- Contained data files – embeds data files in your Python program as data constants so cyber criminals can’t modify them to tamper with your code.
- Plugin support – lets you include an extension to modify how Nuitka compiles your Python code.
Pros:
- Actively maintained with regular updates, patches, and improvements.
- Comprehensive protection features make Nuitka a highly secure compiler.
- Flexibility in modifying options and builds for a specific operating system.
- Code optimization during compilation for better performance.
Cons:
- Cross-compiling is not possible, meaning you can only run compiled executables on the operating system for which they were intended.
- Relies on the CPython runtime interpreter and libraries because Nuitka is incompatible with other alternatives like PyPy or Jython.
6. Codon
If you’re looking for an alternative to CPython with similar syntax, semantics, and libraries, Codon may be a good option, especially for scientific and numerical tasks.
Codon eliminates runtime overhead, offering performance that is up to 100 times better than CPython in single-threaded execution. And unlike vanilla Python, it also supports multithreading.
Key features:
- Static type system – performs runtime type checking during compilation to remove overhead and improve execution efficiency.
- Multithreaded execution – enables your code to run concurrently on multiple cores to improve performance.
- Automatic memory management – handles memory allocation and garbage collection during runtime, offloading this task from your development process.
- Aggressive code optimization – applies techniques like inlining and fusion to significantly improve your code efficiency.
Pros:
- High performance and compatibility with multithreaded execution.
- The learning curve for CPython users is relatively shallow, because it is so similar.
- Interoperability with various Python versions, libraries, packages, and ecosystems.
- Extensive hardware compatibility with multi-core CPUs and GPUs.
Cons:
- Not a drop-in replacement for CPython due to its static type compiling.
- Aggressive optimization results in code that is difficult to debug and traceback.
7. Numba
Numba is a Python-to-machine code JIT compiler that uses the popular LLVM library. It results in highly efficient code that approaches the speed of C or FORTRAN.
Numba is compatible with various operating systems, such as Windows, Linux, macOS, and BSD. It also supports different CPU architectures, including ARM and x86.
Key features:
- Decorator-based optimization – lets you add decorators like @jit to modify your code’s behavior at runtime more easily without modifying the main logic.
- NumPy integration – works seamlessly with NumPy’s arrays and functions, making it suitable for large numerical calculations in data science.
- GPU acceleration – compatible with NVIDIA CUDA, which lets you execute your code on a GPU for a significant performance boost.
- Parallel computation – supports multi-threaded CPU processing and automatic vectorization for more efficient computation.
Pros:
- Easy to learn, given that Numba uses Python syntax with additional decorators.
- Eliminates the need for C/C++ functions as Numba can offer the same performance with Python.
- Compatible with various other programs, like Jupyter Notebook and Dask execution framework.
Cons:
- Niche application, as it is mostly beneficial for numerical computing in data science.
- May create overheads in certain scenarios, especially during initial execution.
8. Taichi
Taichi is a free and open-source JIT compiler that translates Python functions into CPU and GPU machine code. It is known for parallel execution and high performance, making it widely used for resource-intensive numerical computations.
Taichi is suitable for tasks such as real-time physics simulation, robotics, visual effects, and artificial intelligence development.
Key features:
- Interoperability with Python – uses the same syntax and integrates well into the Python ecosystem, supporting various libraries like NumPy.
- SNode – structures data in a flexible way, allowing you to experiment easily with different memory layouts for performance optimization without rewriting code.
- Spatially sparse data structures – in simulations, it computes only relevant areas that contain actual data and ignores empty spaces to optimize memory use.
- Differentiable programming – automatically generates gradients, which are variations in output variables based on input, for a more efficient simulation.
Pros:
- Supports parallel computing for a high-performance compilation.
- Vast compatibility with different GPU backends, like Vulkan and CUDA.
- The repository is open-source and actively maintained.
- Easy to learn given syntax’s similarity to Python.
Cons:
- Limited use in traditional web development – it is mostly used in simulation and machine learning.
- Fewer resources and lower popularity than deep-learning platforms such as TensorFlow.
How to choose the right Python compiler for you
With many Python compilers available offering different features and benefits, choosing one might be tricky. To help you make an informed decision, consider the following criteria:
- Performance – choose a Python compiler that converts your code into a more efficient language, like C/C++ or bytecode. Also, if you want faster and more dynamic compilation at runtime, opt for a JIT compiler.
- Compatibility – ensure your chosen compiler is compatible with your Python version, libraries, and extensions.
- Usability – consider the compiler’s documentation and availability of community support to simplify the learning process. And choose one that you can set up easily.
- Features – pick a compiler with comprehensive features that match your development tasks. For instance, consider extra functionality like debugging support and integration with Python’s IDE.
Remember that the best compiler depends on your needs and use case – Python has very diverse applications.
For example, Taichi is suitable for users who use Python for intensive numerical calculations and simulations but not general web developers.
Hostinger Horizons: an alternative to using a Python compiler
Compilers are crucial to ensure your code runs properly and optimally, but choosing and using one can be tricky, especially if you’re a beginner with minimal technical knowledge.
If you’re just looking to create a simple Python application, a no-code platform like Hostinger Horizons may be an easier option. It offers a graphical user interface and an AI-driven development process, making it suitable for beginners or more seasoned developers.
Using simple prompts, you can design your application’s user interface, add features, and get help integrating a back-end database service. You can also ask Hostinger Horizons to automatically fix errors, or to develop your app using specific technologies or frameworks.
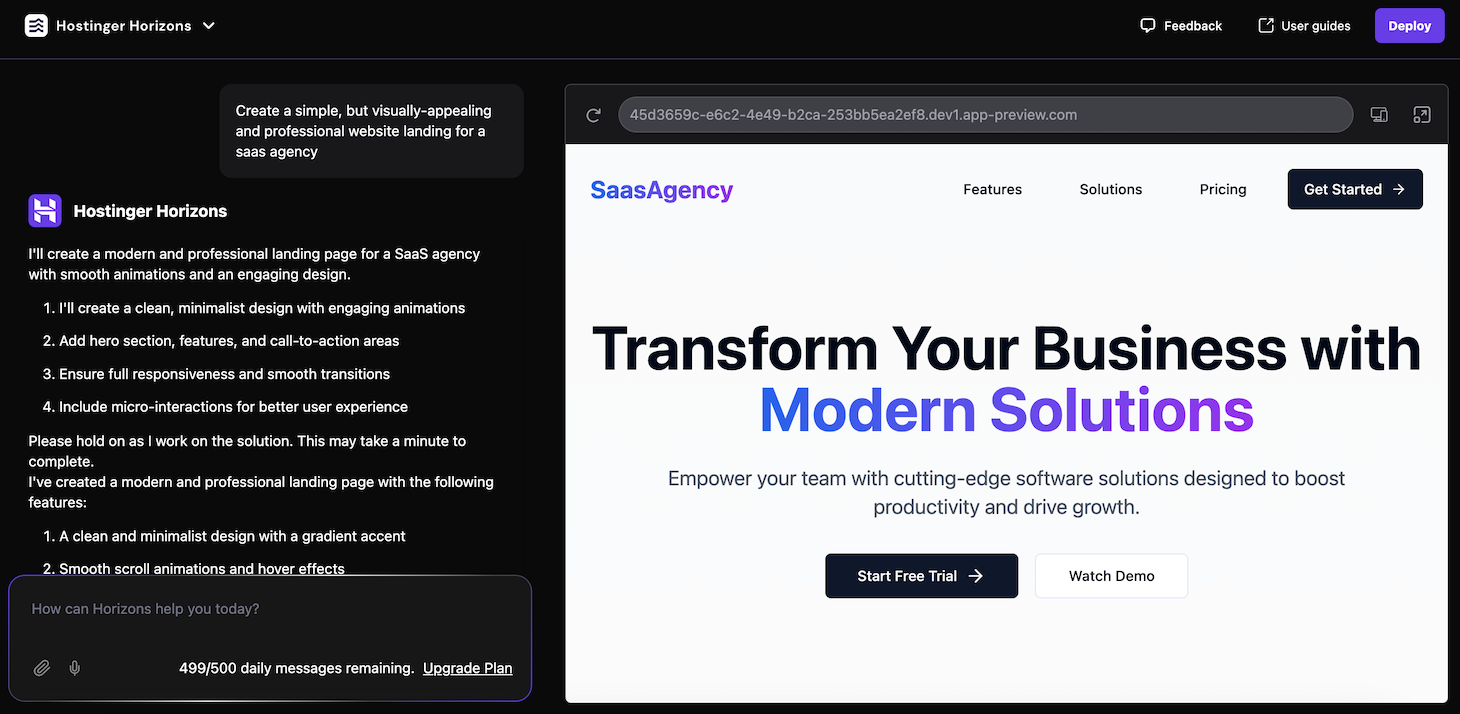
Moreover, Hostinger Horizons lets you preview and interact with your application in real time, streamlining the debugging process. You can then deploy your project in one click with the included Business web hosting plan.
To see this platform in action, check out our article on building a web application using Hostinger Horizons.

Conclusion
Although Python is an interpreted programming language, you might need a compiler to convert it to other languages that are more efficient. Additionally, various compilers offer features that may benefit your specific web development task.
In this tutorial, we have explored the eight best Python compilers. Our top picks are:
- CPython – the most widely used Python compiler with extensive compatibility and functionality.
- Cython – an excellent option for compiling Python into C/C++, offering seamless integration and in-depth memory management.
- Nuitka – an ideal Python compiler for creating a portable and encrypted standalone executable.
- Numba – the best Python compiler for numerical computation, providing GPU-accelerated parallel execution and NumPy integration.
When choosing a Python compiler, make sure it offers the right features for your needs, supports your Python development environment, and provides ample documentation to simplify learning.
Best Python compilers FAQ
How do Python compilers differ from Python interpreters?
Both compilers and interpreters convert Python code into a specific programming language. The difference lies in how they function.
A compiler analyzes the code in its entirety before the program runs, then displays errors afterward. Meanwhile, interpreters work at runtime and operate line by line. Compilers are slower, but the resulting code runs faster.
Can using a Python compiler improve my code?
Yes, a compiler can optimize your code and make it more efficient. This is mainly because compilers can convert your Python code into lower-level languages that execute faster.
For example, converting Python into bytecode lets you skip the parsing process. And translating it to C/C++ removes the interpreting overhead.
What is a good alternative to Python compilers?
Interpreters are good alternatives to compilers, but it depends on your needs. Because interpreters execute code line-by-line, they can speed up debugging and troubleshooting. Interpreters also allow for modification at runtime and produce a smaller file.
However, interpreters produce slower code, which is unsuitable if you prioritize performance, especially on large-scale applications.