8 best JavaScript compilers to use
JavaScript isn’t traditionally a programming language that relies on a compiler like C++ or Java. Instead, it was initially an interpreted language, meaning it executed code line by line at runtime.
But as web apps became more complex, modern JavaScript engines introduced Just-In-Time (JIT) compilation. This enables JavaScript code to be compiled at runtime, improving execution speed.
In this article, we’ll explore the best JavaScript compilers available. We’ll cover their pros and cons, key features, and capabilities to help you choose the right one for your needs.
What is a JavaScript compiler?
A JavaScript compiler transforms modern JS code so that it runs more smoothly in all environments. It improves browser compatibility while optimizing performance.
This allows web developers to use the latest language features without waiting for full browser support. They can incorporate next-generation syntax and functionality with the assurance that their code will run on various platforms.
During the build process, compilers also optimize code by removing unused (dead) code and applying techniques like scope hoisting. These optimizations make the code more suitable for production deployments.
The difference between traditional and JavaScript compilers
Traditional compilers, like those for Java or C++, translate source code into machine code before execution – known as ahead-of-time (AOT) compilation. In contrast, JavaScript compilers act as transpilers, parsing modern JavaScript syntax into earlier versions. Many JavaScript engines use JIT compilation, compiling code at runtime to optimize performance based on actual usage patterns while preserving JavaScript’s dynamic nature.
How does a JS compiler work?
A JavaScript compiler performs one or more key functions: transpiling, minifying, and/or bundling. Each serves a different purpose.
Transpiling
Transpilation converts existing JavaScript code written with newer syntax and features (such as ES6 and beyond) into an older version (like ES5). This ensures compatibility with a wider range of web browsers.
Minifying
Minification reduces JavaScript file sizes by removing unnecessary characters, such as comments, whitespace, and redundant code, without altering functionality. Smaller file sizes lead to faster load times and better performance.
Bundling
Bundling merges multiple JavaScript files and modules into a single file, or at most a few files. This reduces the number of HTTP requests needed to load a web page, resulting in quicker page loading time.
8 best JavaScript compilers
This section lists the top JavaScript compilers that optimize performance across different browsers. We’ve highlighted their standout features, unique capabilities, and potential limitations so you can make an informed decision.
1. TypeScript

TypeScript is an open-source programming language developed by Microsoft. It extends JavaScript with static typing and compiles to plain JavaScript, ensuring compatibility with various browsers and platforms.
Adding optional static types, TypeScript improves code quality and maintainability, making it especially useful for large-scale web applications.
Key features:
- Static typing – lets developers define variable and function types, enabling compile-time error detection and reducing runtime errors.
- Type inference – automatically determines types when they are not explicitly stated.
- Interfaces – defines contracts within the code, ensuring consistency across different components.
- Classes and modules – supports object-oriented programming principles, including classes, inheritance, and modules.
Pros:
- Enhances code quality with static typing and early error detection.
- Integrates seamlessly with existing JavaScript codebases and libraries.
- Provides a vast collection of type definitions and third-party tools.
Cons:
- JavaScript developers may need time to adapt to TypeScript’s type system and additional features.
- Configuring TypeScript in existing JavaScript projects requires extra tooling and build process adjustments.
2. Babel
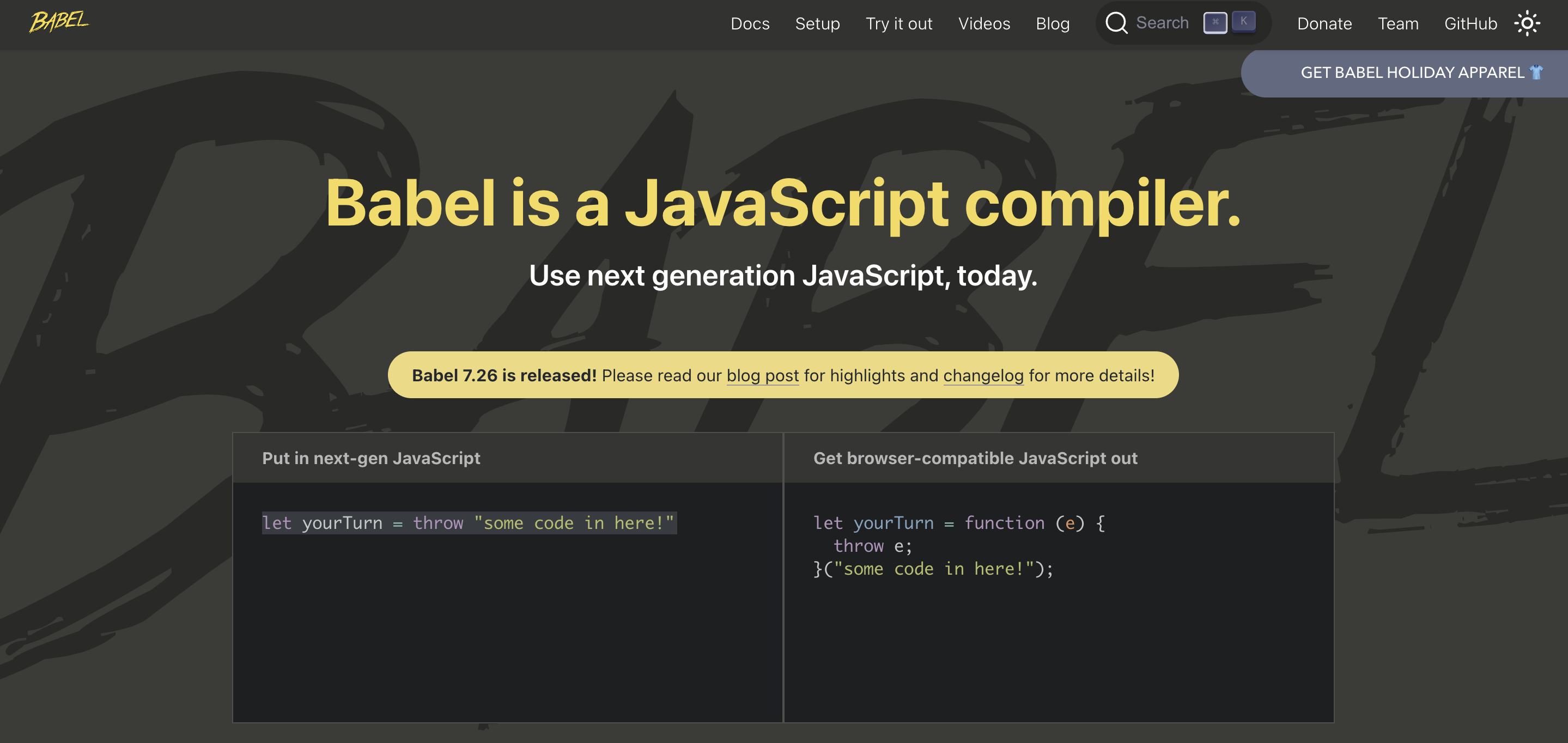
Babel is a widely used open-source JavaScript transpiler that lets developers write next-gen JavaScript while ensuring compatibility with older browsers and environments.
By transpiling ECMAScript 2015+ (ES6+) syntax into backward-compatible JavaScript, Babel enables developers to use the latest features without sacrificing support for legacy systems.
Key features:
- Transpilation of modern syntax – converts new JavaScript features, such as arrow functions and classes, into ES5 syntax for broader compatibility.
- Plugin and preset ecosystem – provides numerous plugins and presets that extend functionality, supporting JSX, TypeScript, and experimental JavaScript proposals.
- Automatic polyfilling – integrates with tools like core-js to include polyfills for unsupported features, such as Promise or Array.from.
- Integration with build tools – works seamlessly with bundlers and task runners like Webpack, Gulp, and Grunt.
Pros:
- Ensures recent JavaScript features work in older browsers, broadening compatibility.
- Has a large and active community that provides continuous updates and comprehensive documentation.
Cons:
- The transpilation process can introduce performance overhead, slowing down build times.
- Configuring Babel with the right presets and plugins can be complicated for beginners.
3. CoffeeScript
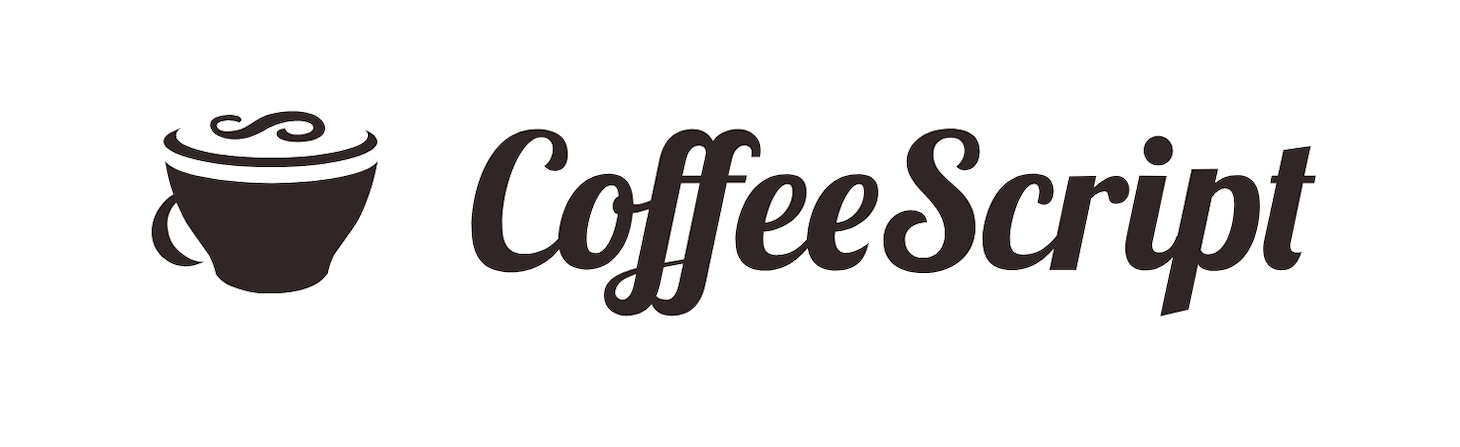
CoffeeScript is a programming language that compiles to JavaScript. It is mainly developed to be a simpler alternative to JS, with shorter syntax inspired by Ruby and Heskell.
In addition to syntax improvements, CoffeeScript introduces an auto-scoping feature to avoid variable overwriting, which can otherwise cause errors at runtime.
Key features:
- Auto-scoping – automatically assigns a variable to a specific function as written in the code, preventing it from overlapping with the global variable.
- Efficient syntax – removes bloat characters from the JS syntax like parentheses, braces, and semicolons to make the code less verbose.
- Implicit return – allows functions to automatically return the last expression without needing the return function, making the code easier to follow.
- List comprehension – enables you to create or manipulate arrays from an existing list to make data iteration more concise and organized.
Pros:
- CoffeeScript’s shorter and less verbose syntax is easier to learn for beginners than JS.
- Given its conciseness, CoffeeScript code is more efficient and quicker to compile than JS.
- Compatible with any JavaScript runtime environment or web browser compilers.
- CoffeeScript code is easily maintainable because of its concise and easily readable syntax.&
Cons:
- CoffeeScript code is only debuggable as JavaScript unless checked manually.&
- Requires JavaScript to learn due to their similar logic.
- Declining in popularity, as modern JS has adopted some similar features, so there are fewer resources available.
4. ReScript
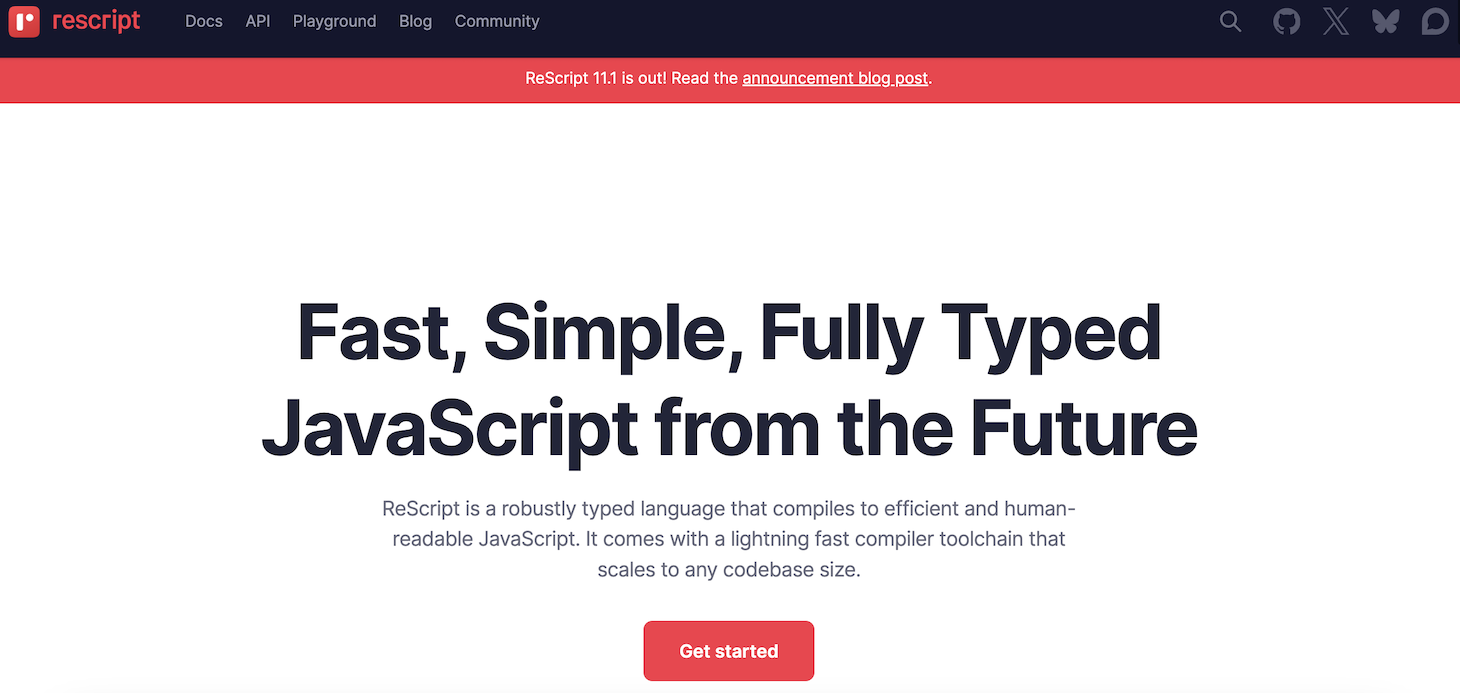
ReScript is a rebrand of BuckleScript – a JavaScript compiler for OCaml. It aims to provide the interoperability of JS while offering OCaml’s robust type system, which lets you catch errors before runtime.
ReScript is also known for its fast build time, which is mainly due to its efficient Ninja build system and JS wrappers.
Key features:
- Robust static type checking – ensures all types are defined during compiling and encourages the use of simpler types instead of abstract ones.
- JavaScript ecosystem integration – works with existing JavaScript codebase and libraries, and is easily reversible to plain JS.
- Pattern matching – provides conditional logic using patterns, which is clearer and more reliable than if-else statements.
- Immutable data structure – disallows modification of values in your code after creation to maintain consistency and simplify debugging.
Pros:
- Easy debugging with human-readable JS syntax and source map.
- Comprehensive solution with various built-in tooling features, including a package manager and a code prettifier.
- Incremental builds only recompile files that change, shortening the overall build time.
Cons:
- Typing is less flexible than other compilers like Babel and TypeScript.
- ReScript doesn’t offer that much improvement over vanilla JS in terms of verbosity and simplicity.
5. SWC
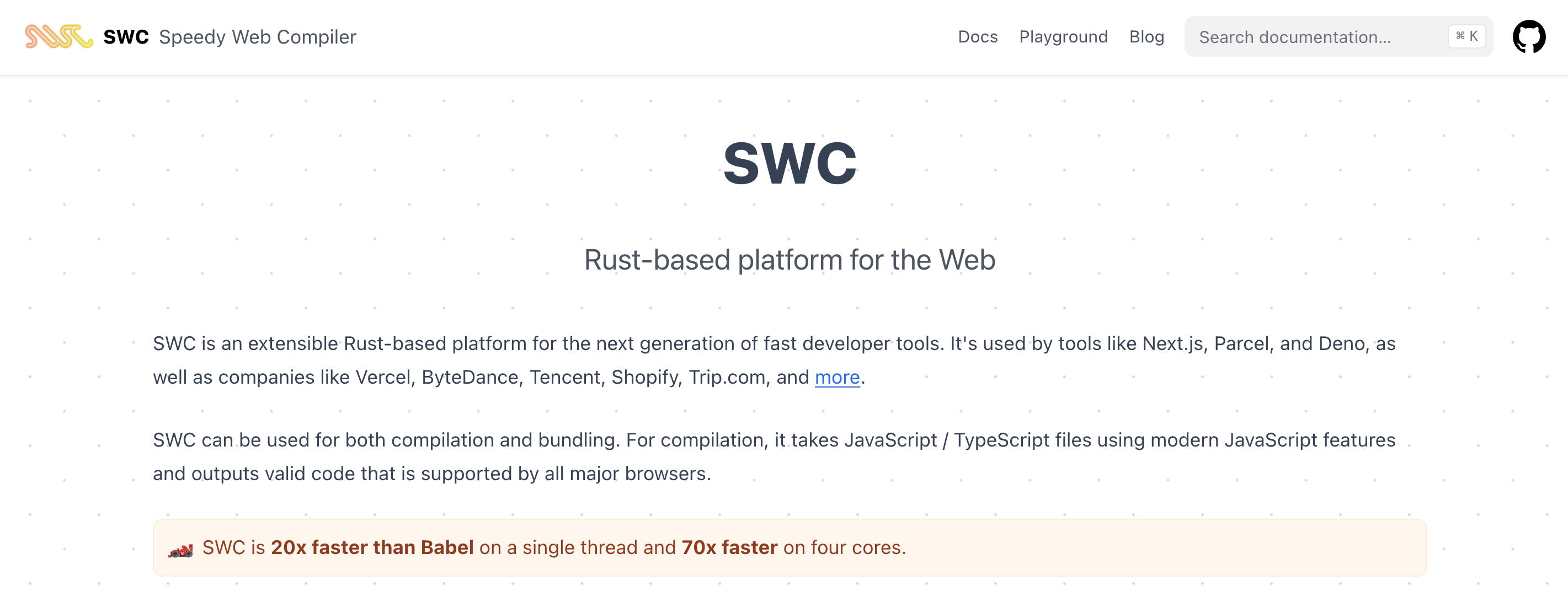
Written in Rust, SWC (Speedy Web Compiler) is an open-source JavaScript and TypeScript compiler built for high performance. It provides rapid compilation and transformation for both languages.
SWC often outperforms traditional tools like Babel, making it especially beneficial for large-scale projects.
Key features:
- Native TypeScript support – offers built-in support for TypeScript, eliminating the need for additional plugins or configurations.
- Minimal configuration – offers a simpler setup than alternatives, like Babel.
- Integration with modern frameworks – works seamlessly with Next.js, Vite, and Webpack.
Pros:
- SWC’s Rust-based architecture compiles code rapidly, significantly reducing build times.
Cons:
- SWC has a relatively small plugin ecosystem, limiting customization options.
- Offers fewer resources and less community support than long-standing tools like Babel.
6. AssemblyScript
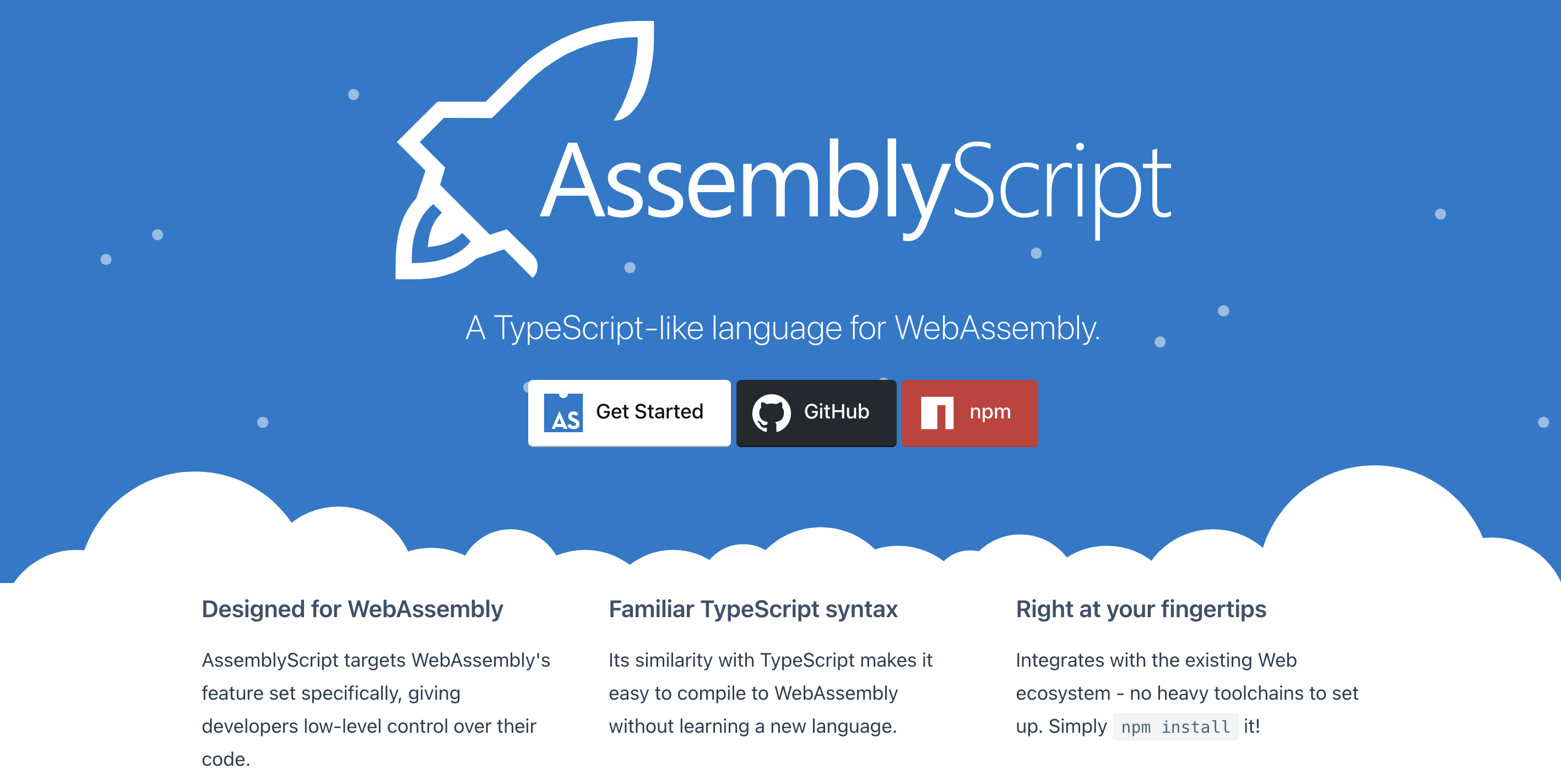
AssemblyScript is an open-source programming language that compiles a statically typed subset of TypeScript into WebAssembly (Wasm).
Leveraging WebAssembly’s performance benefits, AssemblyScript lets developers familiar with TypeScript and JavaScript write code for web browsers, server-side applications, and other Wasm-supported environments.
Key features:
- Optimized for WebAssembly – provides low-level control over code execution and enables performance optimizations.
- Seamless integration with JavaScript – integrates AssemblyScript modules into existing JavaScript projects.
- Portable compiler – supports both command-line and programmatic usage for flexible deployment.
Pros:
- Developers familiar with TypeScript or JavaScript can quickly adopt AssemblyScript due to its similar syntax.
- Compiling to WebAssembly enables near-native execution speeds, making it ideal for performance-critical projects.
Cons:
- Omits certain TypeScript and JavaScript features, requiring developers to adjust their coding practices.
- Relies on Node.js and npm, which may not align with all project environments or deployment targets.
7. Traceur
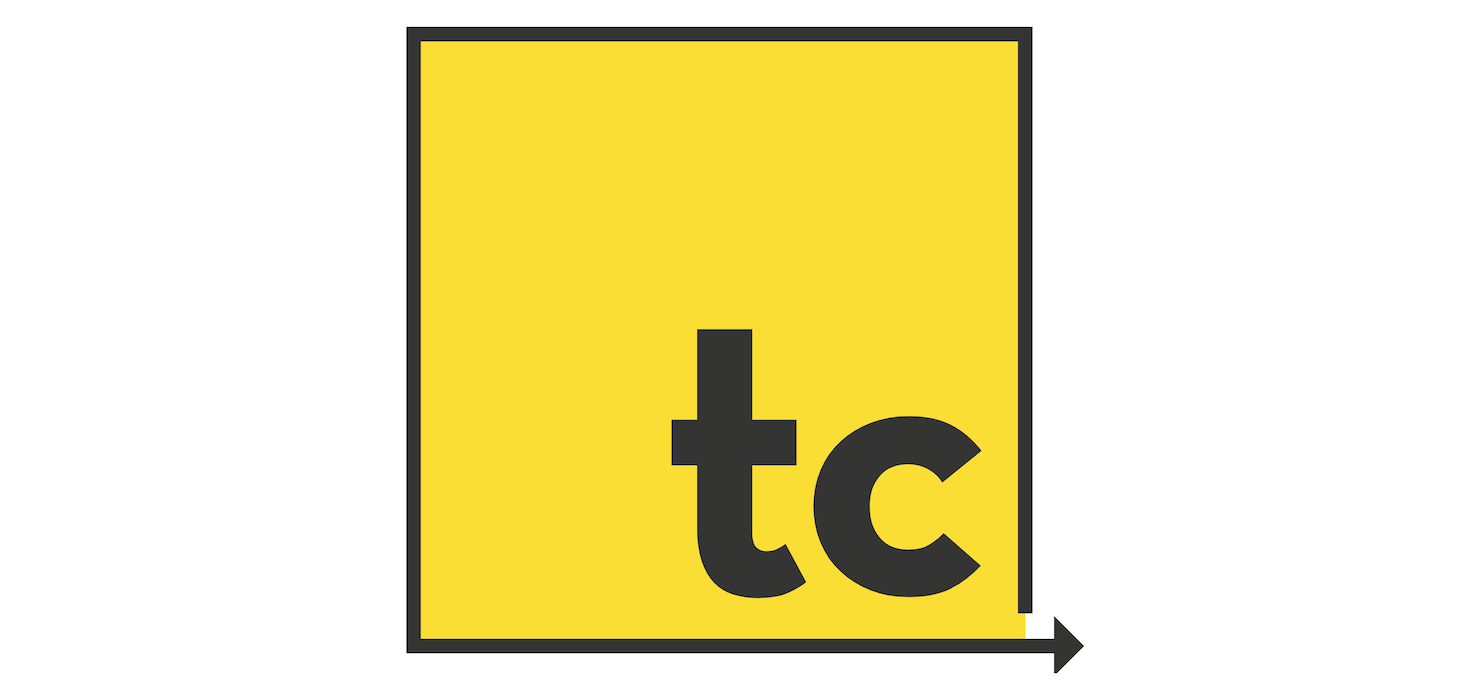
Traceur is a JavaScript compiler mainly known for its ability to maintain compatibility between different JS versions. It is an excellent alternative to Babel, especially if you want a polyfill library.
You can compile JavaScript to an older version and fine-tune the configuration to ensure it runs in a runtime environment that doesn’t natively support the newer release.
Key features:
- Backward source map – lets you map a newer version of JavaScript with the older one during debugging.
- Polyfill libraries – polyfills can help to ensure your code will run on older browsers or devices.
- Feature selector – enables you to remove modern JavaScript features when compiling your code to an older version, further ensuring compatibility.
- Asynchronous execution – allows you to use the await and async functions to enable non-blocking operation, which is not supported on legacy browsers by default.
Pros:
- Compatibility with various JavaScript versions, browsers, and runtime environments.
- Simplifies debugging JavaScript across different versions.
- Integration with various buildpacks, like Grunt, Glup, and WebPack.
Cons:
- Transpiling legacy JavaScript adds a performance overhead due to extra libraries and polyfills.
- Setting up Traceur can be complicated, especially due to its high capacity for customization.
- Traceur’s documentation and community are relatively limited, making learning difficult.
8. ClojureScript
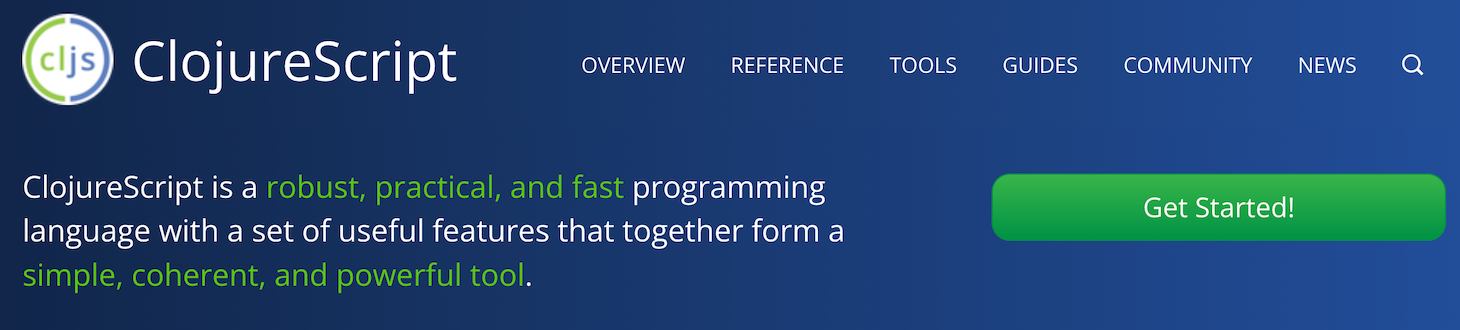
ClojureScript is a JavaScript compiler for Clojure, known for its interactive development experience and immutable data structures. These immutable data enable concurrency and asynchronous processing, complementing JavaScript’s event-driven model.
Plus, as a dialect of Lisp, ClojureScript lets you extend your code syntax for complex and specific tasks.
Key features:
- Macros – allows users to create a function that generates new code during compilation to reduce runtime overhead and boilerplates.
- Data consistency – provides an immutable and persistent data structure, which enables concurrency and improves code safety.
- Pure functional paradigm – uses pure functions and declarative programming to achieve more predictable code behavior, which results in simpler troubleshooting.
- Hot code reloading – enables your application preview to reflect code changes in near-real time without restarting.
Pros:
- Easy rapid prototyping with REPL-driven development and hot reloading, making it ideal for web designers.
- Suitable for complex data logic for its macros and extendable syntax.
- Seamless interaction with JavaScript, JS frameworks, and the npm ecosystem.
Cons:
- Limited ecosystem due to its relatively low popularity and niche language.
- As a List dialect, ClojureScript’s syntax is complicated and may be difficult to understand.
Alternative to coding with JavaScript: Hostinger Horizons
A compiler is an important tool for developing a JavaScript-based web application, to ensure your code runs properly in a range of browsers. However, beginners with minimal technical experience might find it difficult to choose and use a compiler.
Fortunately, there are other ways to create a web application that are more beginner-friendly. If you wish to create a simple app that doesn’t require complex features and down-to-the-code customization, a no-code AI platform like Hostinger Horizons might be a suitable solution.
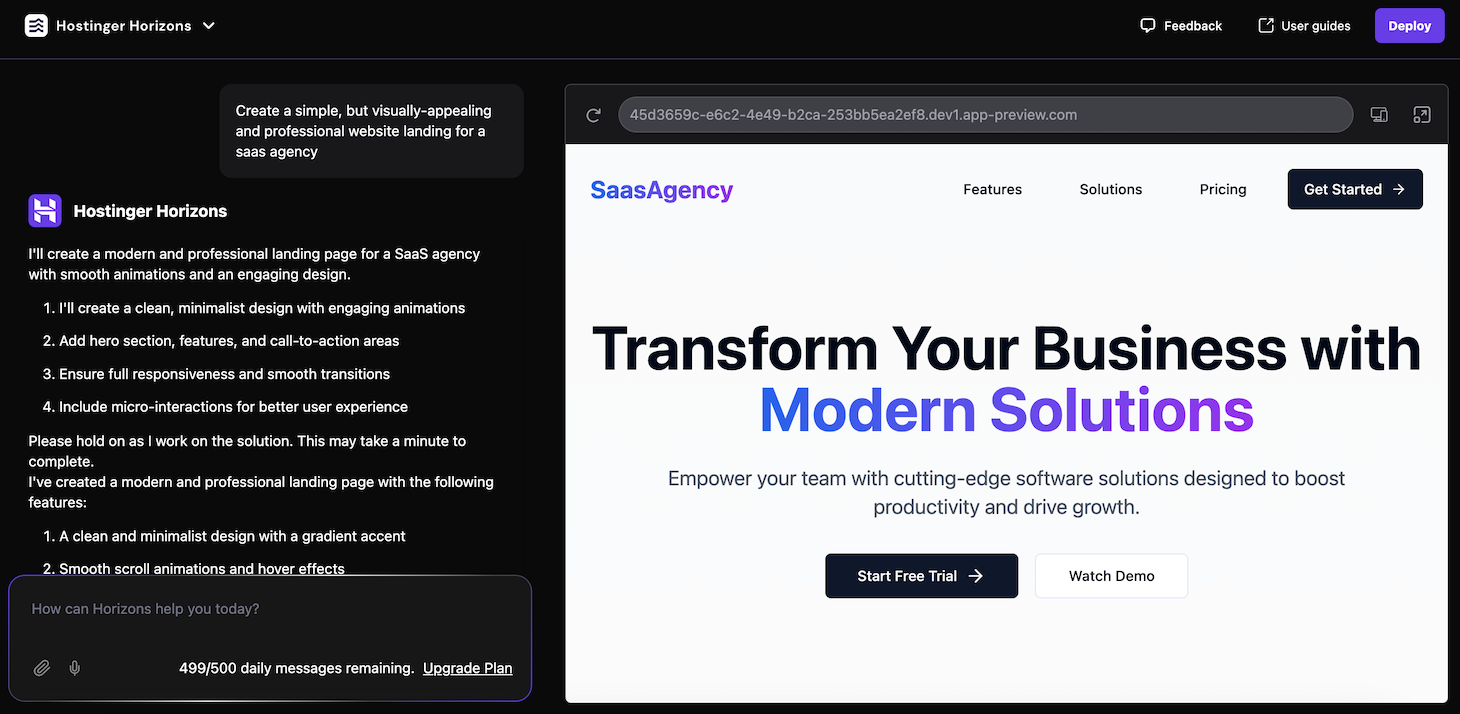
You can build a fully functional web application using Hostinger Horizons by simply writing prompts. From designing the user interface to integrating a back-end database service, this platform can manage the entire development process.
From the same dashboard, you can preview and interact with your application in real time, streamlining the debugging process. When ready, you can deploy your application in one click using the included Business web hosting plan.
Hostinger Horizons’ intuitive interface and no-code development experience make it an ideal tool for beginners or solopreneurs. If you want to learn more about this platform, read our tutorial on getting started with Hostinger Horizons.

Conclusion
Compilers ensure your JavaScript web application runs properly in different browsers and runtime environments. They also provide additional benefits, such as performance and maintainability improvements.
In this article, we have explored the eight best JavaScript compilers by highlighting their features and advantages. Among them, our top picks are:
- TypeScript – the best overall JS compiler with extensive support and integration.
- Babel – an excellent cross-version JS compiler offering backward compatibility with older versions and legacy environments.
- CoffeeScript – an ideal JS compiler if you want to write code in simpler and more efficient syntax.
- ReScript – a suitable JS compiler if you are looking for quick build time and built-in development tooling.
If you are developing a simple JavaScript application and are finding it too time-consuming to write the code from scratch, don’t forget to consider Hostinger Horizons. It lets you create a functional application using simple AI prompts that you can launch with a single click.
Best JavaScript compilers FAQ
How does a JavaScript compiler differ from a bundler?
A JavaScript compiler converts modern JS code into a version that works across different environments, often adding static typing or new syntax support. In contrast, a bundler merges multiple JS files and their dependencies into a single file, reducing the number of HTTP requests and improving webpage load times.
What factors should I consider when choosing a JavaScript compiler?
When selecting a JavaScript compiler, consider project requirements, desired language features, compatibility with existing codebases, performance impact, community support, and available tooling. The best choice should align with your development workflow.
What is a good alternative to a JS compiler?
There are several alternatives to a JavaScript compiler, but if you’re a beginner and want to avoid browser compatibility issues, try Hostinger Horizons. It’s not a direct replacement, but it lets you generate code using the power of AI. Just describe the features and interface you want, and it will create a ready-to-launch web app with all the underlying code in place